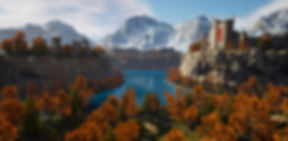
Prime Number Algorithm
C# / Unity
using System.Globalization;
using UnityEngine;
using TMPro;
public class PrimeNumberChecker : MonoBehaviour
{
public TextMeshProUGUI outputText;
private TMP_InputField textInputField;
private int intInput;
private void Start()
{
textInputField = GetComponent<TMP_InputField>();
}
private void Update()
{
if (Input.GetKeyDown(KeyCode.Return))
{
//Check if a number was inputted or not
if (int.TryParse(textInputField.text, NumberStyles.Number, CultureInfo.CurrentCulture.NumberFormat, out intInput))
{
//Call function to test for prime or not
if (CheckInput(intInput))
{
outputText.text = "'" + intInput.ToString("#,##0") + "' \nIS a prime number!";
}
else
{
outputText.text = "'" + intInput.ToString("#,##0") + "' \nIs NOT a prime number!";
}
}
//Tell user they didn't enter a number
else
{
outputText.text = "No int (Number) was entered. \nOr int too large.";
}
//Reset input field
textInputField.text = "";
}
}
public bool CheckInput(int input)
{
//Get the modulo (remainder) to check if prime
int remainderNum;
//Loop through all possible numbers
for(int i = (input - 1); i > 0; i--)
{
//Set remainder to modulo of original number and current loop iteration
remainderNum = input % i;
//End loop and return false if any number other than 1 has no remainder
if(remainderNum == 0 && i != 1)
{
return false;
}
}
//Return true since no number can be divided evenly
return true;
}
}